1 # colors.js [](https://travis-ci.org/Marak/colors.js)
3 ## get color and style in your node.js console
5 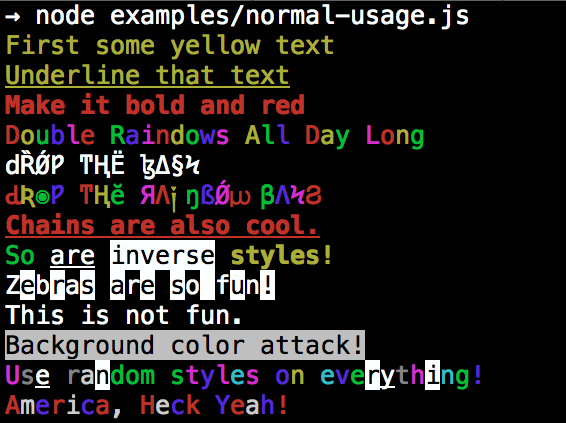
59 By popular demand, `colors` now ships with two types of usages!
64 var colors = require('colors');
66 console.log('hello'.green); // outputs green text
67 console.log('i like cake and pies'.underline.red) // outputs red underlined text
68 console.log('inverse the color'.inverse); // inverses the color
69 console.log('OMG Rainbows!'.rainbow); // rainbow
70 console.log('Run the trap'.trap); // Drops the bass
74 or a slightly less nifty way which doesn't extend `String.prototype`
77 var colors = require('colors/safe');
79 console.log(colors.green('hello')); // outputs green text
80 console.log(colors.red.underline('i like cake and pies')) // outputs red underlined text
81 console.log(colors.inverse('inverse the color')); // inverses the color
82 console.log(colors.rainbow('OMG Rainbows!')); // rainbow
83 console.log(colors.trap('Run the trap')); // Drops the bass
87 I prefer the first way. Some people seem to be afraid of extending `String.prototype` and prefer the second way.
89 If you are writing good code you will never have an issue with the first approach. If you really don't want to touch `String.prototype`, the second usage will not touch `String` native object.
93 To disable colors you can pass the following arguments in the command line to your application:
96 node myapp.js --no-color
99 ## Console.log [string substitution](http://nodejs.org/docs/latest/api/console.html#console_console_log_data)
103 console.log(colors.green('Hello %s'), name);
104 // outputs -> 'Hello Marak'
109 ### Using standard API
113 var colors = require('colors');
129 console.log("this is an error".error);
131 // outputs yellow text
132 console.log("this is a warning".warn);
135 ### Using string safe API
138 var colors = require('colors/safe');
140 // set single property
141 var error = colors.red;
142 error('this is red');
159 console.log(colors.error("this is an error"));
161 // outputs yellow text
162 console.log(colors.warn("this is a warning"));
166 You can also combine them:
169 var colors = require('colors');
172 custom: ['red', 'underline']
175 console.log('test'.custom);
178 *Protip: There is a secret undocumented style in `colors`. If you find the style you can summon him.*